Using the Texture
Well now that you have your texture uploaded you want to do something with it since its useless just sitting in memory. The process for applying a texture to geometry greatly depends on what type of data you are dealing with and how you would like things to run. Due to this fact in this section I will relay you some pointers on texture mapping, give an example of how to texture a quad and explain the texture coordinate system.
Pointers |
- Make sure that you have enabled texturing. You do this with the glEnable (GL_TEXTURE_2D) call.
- Make sure that you bind to a texture before you do any sort of glBegin/glEnd. You cannot bind to a texture in the middle of a begin/end pair.
- Make sure that you specify a texture coordinate before each vertex that makes up a face. If you have 3 verticies the pattern for texture mapping the triangle would go like this: TexCoord; VertexCoord; TexCoord; VertexCoord; TexCoord; VertexCoord;
- Make sure that you store your texture id's in variables, it makes things easier.
- Make use of glGenTextures. Its the easy way to get a free texture id.
|
A Textured Quad |
Below is an example of how you would texture a quad. This code assumes that texturing has been enabled and that there has been a texture uploaded with the id of 13.
glBindTexture (GL_TEXTURE_2D, 13);
glBegin (GL_QUADS);
glTexCoord2f (0.0, 0.0);
glVertex3f (0.0, 0.0, 0.0);
glTexCoord2f (1.0, 0.0);
glVertex3f (10.0, 0.0, 0.0);
glTexCoord2f (1.0, 1.0);
glVertex3f (10.0, 10.0, 0.0);
glTexCoord2f (0.0, 1.0);
glVertex3f (0.0, 10.0, 0.0);
glEnd ();
|
The Texture Coordinate System |
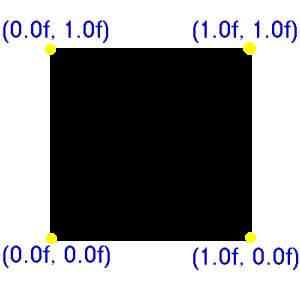
The image above shows the OpenGL texture coordinate system. In the code above the calls to glTexCoord2f are very important as to what the end result of the texture mapping will be. When you make a call to glTexCoord2f (x,y) OpenGL places the texture coordinate at that place on the image. If you are texturing a triangle there will be three texture coords on the image. Once a glEnd is reached the triangle which is formed by the texture coordinates is then mapped onto the triangle that is made up from the verticies.
|
|